The AND or conjunction operator, according to Boole, outputs “true” only when both inputs are “true.” That is, it works like this:
Input 1
Input 2
Output
false
false
false
false
true
false
true
false
false
true
true
true
If you gave the Boolean operator AND a first input of “false” and a second input of “true,” it would output “false.”
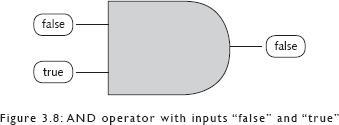
The output of “(Teddy can fly) AND (Teddy is a dog)” would therefore be “false.” But the output of “(Teddy is a dog) AND (Teddy has a keen sense of smell)” would be “true.”
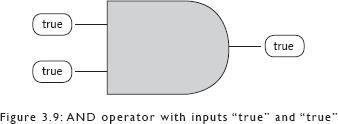
Other operators work similarly. The “truth table” for the Boolean OR operator would look like this:
Input 1
Input 2
Output
false
false
false
false
true
true
true
false
true
true
true
true
So, the output of “(Teddy can fly) OR (Teddy is a dog)” would be “true.” That is, a first input of “false” and a second input of “true” would produce the output “true.”
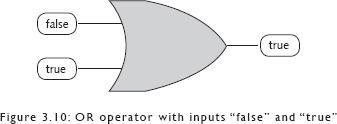
If one were to adopt the convention that “false” was represented by the digit “0” and “true” by “1,” the functioning of the OR operator could be represented as follows:
Input 1
Input 2
Output
0
0
0
0
1
1
1
0
1
1
1
1
And so we could draw our OR operator example like this:
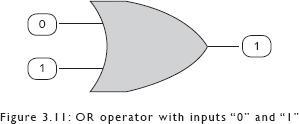
The XOR operator — sometimes referred to as the “exclusive-OR” operator — is a variation of the OR operator. It outputs “true” if either, but not both, of the inputs are “true.”
Input 1
Input 2
Output
0
0
0
0
1
1
1
0
1
1
1
0
You can think of XOR as an “either-or” operator — it returns “true” if one of the inputs is true, but returns “false” if both of the inputs are “true” or if both of the inputs are “false.” For example, a future robot-run restaurant might use an XOR operation to test whether your sandwich order was valid: “(With soup) XOR (With salad)”—you could have soup or salad, but not both or nothing. The last line of the truth table for the XOR operator could be drawn like this:
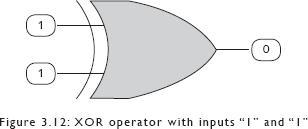
Boolean algebra allows the translation of logical statements into mathematical expressions. By substituting ones and zeros into our soup-XOR-salad statement above, we can get back another number we can use in further operations.
Now here’s the magical part: you can build simple physical objects — logic gates — that mechanically reproduce the workings of Boolean operators. Here is an AND logic gate built out of LEGO brides, cogs, and wheels by Martin Howard, a physicist from the UK:
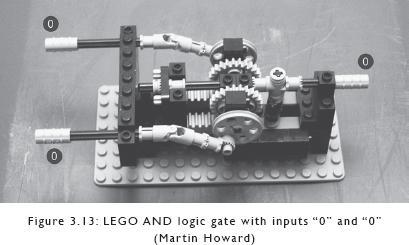
This is a push-pull logic gate. The two levers on the left are for input: a pushed-in lever represents a value of “true” or “1,” while a lever in the “out” position represents a “false” or “0.” The mechanism of the logic gate — its gears and rods — has been set up so that when you push in the input levers on the left, the output lever on the right automatically takes a position (in or out) that follows the workings of the Boolean logical operator AND. In figure 3.14, both input levers have been pushed in (set to “true” and “true”), and so the output lever has slid into a position representing “true” or “1.” Or, in Boolean terms, “true AND true = true.” Any possible positioning of the input levers will produce the correct positioning of the output lever. The logic gate always follows the truth table for the AND operator.
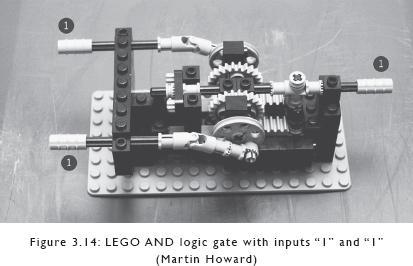
And here is a push-pull XOR logic gate that mimics the workings of the Boolean XOR operator:
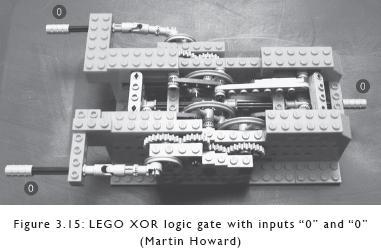
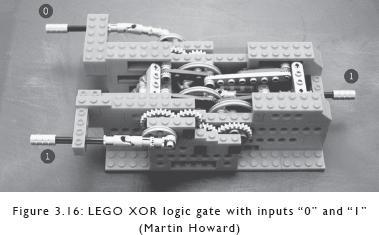
If you’re still having trouble visualizing how these LEGO logic gates work, you can watch videos at http://www.randomwraith.com/logic.html. A physical logic gate is any device that — through artful construction — can correctly replicate the workings of one of the Boolean logical operators.
You may still be wondering how all of this leads us toward computation, toward calculation. Well, as it happens, you can also represent numbers in a binary fashion, with ones and zeros, or with absence and presence, off states and on states. In binary notation, the decimal number “3” is “11.” How does this work? You’ll recall from elementary school that in the decimal system, the position of a digit — from right to left — changes what that digit means. If you write the decimal number “393,” you are putting the digits into columns like this:
Hundreds
(10
2
)
Tens
(10
1
)
Ones
(10
0
)
3
9
3
So what you’re representing when you write “393” is something like “three hundreds, plus nine tens, plus three ones” or “(3 × 102) + (9 × 101) + (3 × 100).” A more precise way to think about the columns in the decimal system is to say each column, from right to left, represents an increase by a factor of ten. The small superscript number — the exponent — tells you how many times to use the number in a multiplication by itself. So, the “Hundreds” column represents quantities of “102” or “10 × 10.” Any number to the power of 1 gives you the number itself, so “101” is “10”; and any number to the power of zero is just “1,” so “100” is “1.”
In base-2 or binary notation, our column headings would look like this:

And you would write “393” in binary like this:

When you write the binary number “110001001,” you are putting a “1” in every column that you want to include in your reckoning of the quantity you are trying to represent. In a base-2 system, you have only two symbols you can use, “0” and “1” (as opposed to the ten symbols you use in a base-10 system, “0” through “9”). So, with “110001001,” you are representing something like “256, plus 128, plus 8, plus 1” or “(1 × 28) + (1 × 27) + (1 × 23) + (1 × 20)”—which equals decimal “393.”
Decimal “9” is the same as binary “1001,” and decimal “5” is binary “101”—all very baffling to the decimal-using brain, but completely consistent and workable. So if you wanted to add “9” to “5” in binary, it would look like this:
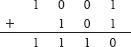
And binary “1110” is of course equivalent to “8 + 4 + 2 + 0” or decimal “14.” From the above, you can deduce the rules of binary addition:
0 + 0 = 0
0 + 1 = 1
1 + 0 = 1
1 + 1 = 0, and carry 1
The last rule may seem a bit mystifying until you recall how addition works in decimal arithmetic. In decimal, you use the digits “0” through “9” to represent numbers; when the sum of the digits in a column exceeds “9” you write the least significant figure (“4” in the number “14”) in that column and carry the more significant figure (“1” in the number “14”) to the next column on the left. In binary notation, you can only use the digits “1” and “0”—so when you add “1” to “1,” you write “0” in that column and carry “1.”